"""
Basic imgae load, plot, resize, etc..
Sungjoon Choi ([email protected])
"""
import numpy as np
import os
from scipy.misc import imread, imresize
import matplotlib.pyplot as plt
import skimage.io
import skimage.transform
%matplotlib inline
print ("Packs loaded")
Packs loaded
cwd = os.getcwd()
print ("Current folder is %s" % (cwd) )
def print_typeshape(img):
print("Type is %s" % (type(img)))
print("Shape is %s" % (img.shape,))
Current folder is /home/enginius/github/tensorflow-101/notebooks
Load & plot
cat = imread(cwd + "/images/cat.jpg")
print_typeshape(cat)
plt.figure(0)
plt.imshow(cat)
plt.title("Original Image with imread")
plt.draw()
Type is <type 'numpy.ndarray'>
Shape is (1026, 1368, 3)
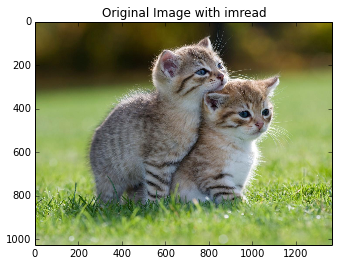
cat2 = imread(cwd + "/images/cat.jpg").astype(np.float)
print_typeshape(cat2)
plt.figure(0)
plt.imshow(cat2)
plt.title("Original Image with imread.astype(np.float)")
plt.draw()
Type is <type 'numpy.ndarray'>
Shape is (1026, 1368, 3)
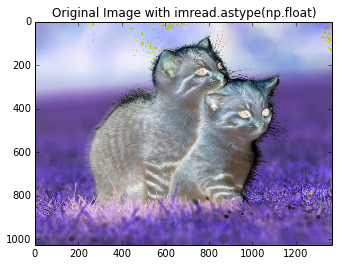
cat3 = imread(cwd + "/images/cat.jpg").astype(np.float)
print_typeshape(cat3)
plt.figure(0)
plt.imshow(cat3/255.)
plt.title("Original Image with imread.astype(np.float)/255.")
plt.draw()
Type is <type 'numpy.ndarray'>
Shape is (1026, 1368, 3)
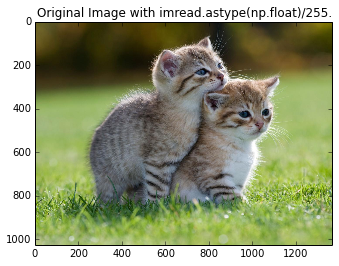
Resize
catsmall = imresize(cat, [100, 100, 3])
print_typeshape(catsmall)
plt.figure(1)
plt.imshow(catsmall)
plt.title("Resized Image")
plt.draw()
Type is <type 'numpy.ndarray'>
Shape is (100, 100, 3)
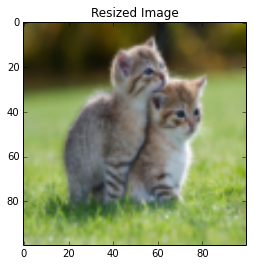
Grayscale
def rgb2gray(rgb):
if len(rgb.shape) is 3:
return np.dot(rgb[...,:3], [0.299, 0.587, 0.114])
else:
print ("Current Image if GRAY!")
return rgb
catsmallgray = rgb2gray(catsmall)
print ("size of catsmallgray is %s" % (catsmallgray.shape,))
print ("type of catsmallgray is", type(catsmallgray))
plt.figure(2)
plt.imshow(catsmallgray, cmap=plt.get_cmap("gray"))
plt.title("[imshow] Gray Image")
plt.colorbar()
plt.draw()
size of catsmallgray is (100, 100)
('type of catsmallgray is', <type 'numpy.ndarray'>)
/usr/lib/pymodules/python2.7/matplotlib/collections.py:548: FutureWarning: elementwise comparison failed; returning scalar instead, but in the future will perform elementwise comparison
if self._edgecolors == 'face':
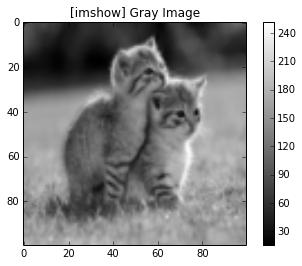
Reshape
catrowvec = np.reshape(catsmallgray, (1, -1));
print ("size of catrowvec is %s" % (catrowvec.shape,))
print ("type of catrowvec is", type(catrowvec))
catmatrix = np.reshape(catrowvec, (100, 100));
print ("size of catmatrix is %s" % (catmatrix.shape,))
print ("type of catmatrix is", type(catmatrix))
size of catrowvec is (1, 10000)
('type of catrowvec is', <type 'numpy.ndarray'>)
size of catmatrix is (100, 100)
('type of catmatrix is', <type 'numpy.ndarray'>)
Load from folder
cwd = os.getcwd()
path = cwd + "/images/cats"
valid_exts = [".jpg",".gif",".png",".tga", ".jpeg"]
print ("%d files in %s" % (len(os.listdir(path)), path))
imgs = []
names = []
for f in os.listdir(path):
ext = os.path.splitext(f)[1]
if ext.lower() not in valid_exts:
continue
fullpath = os.path.join(path,f)
imgs.append(imread(fullpath))
names.append(os.path.splitext(f)[0]+os.path.splitext(f)[1])
print ("%d images loaded" % (len(imgs)))
38 files in /home/enginius/github/tensorflow-101/notebooks/images/cats
38 images loaded
nimgs = len(imgs)
randidx = np.sort(np.random.randint(nimgs, size=3))
print ("Type of 'imgs': ", type(imgs))
print ("Length of 'imgs': ", len(imgs))
for curr_img, curr_name, i \
in zip([imgs[j] for j in randidx]
, [names[j] for j in randidx]
, range(len(randidx))):
print ("[%d] Type of 'curr_img': %s" % (i, type(curr_img)))
print (" Name is: %s" % (curr_name))
print (" Size of 'curr_img': %s" % (curr_img.shape,))
("Type of 'imgs': ", <type 'list'>)
("Length of 'imgs': ", 38)
[0] Type of 'curr_img': <type 'numpy.ndarray'>
Name is: images (19).jpeg
Size of 'curr_img': (246, 205, 3)
[1] Type of 'curr_img': <type 'numpy.ndarray'>
Name is: images (32).jpeg
Size of 'curr_img': (194, 259, 3)
[2] Type of 'curr_img': <type 'numpy.ndarray'>
Name is: images (11).jpeg
Size of 'curr_img': (183, 275, 3)
nimgs = len(imgs)
randidx = np.sort(np.random.randint(nimgs, size=3))
for curr_img, curr_name, i \
in zip([imgs[j] for j in randidx]
, [names[j] for j in randidx], range(len(randidx))):
plt.figure(i)
plt.imshow(curr_img)
plt.title("[" + str(i) + "] ")
plt.draw()
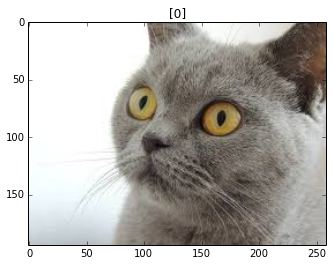
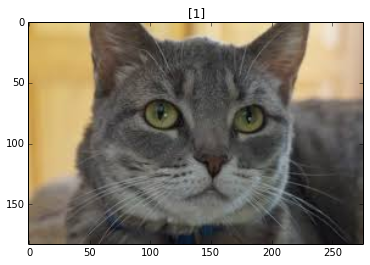
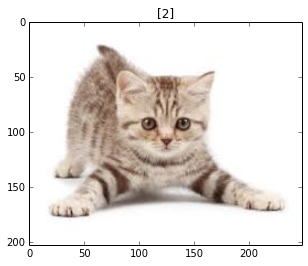
print "That was all!"
That was all!