LOAD PACKAGES!
import numpy as np
print ("Loading package(s)")
Loading package(s)
PRINT function usages
print ("Hello, world")
x = 3;
print ("Integer: %01d, %02d, %03d, %04d, %05d"
% (x, x, x, x, x))
x = 123.456;
print ("Float: %.0f, %.1f, %.2f, %1.2f, %2.2f"
% (x, x, x, x, x))
x = "Hello, world"
print ("String: [%s], [%3s], [%20s]"
% (x, x, x))
Hello, world
Integer: 3, 03, 003, 0003, 00003
Float: 123, 123.5, 123.46, 123.46, 123.46
String: [Hello, world], [Hello, world], [ Hello, world]
FOR + IF/ELSE
dlmethods = ["ANN", "MLP", "CNN", "RNN", "DAE"]
for alg in dlmethods:
if alg in ["ANN", "MLP"]:
print ("We have seen %s" % (alg))
We have seen ANN
We have seen MLP
dlmethods = ["ANN", "MLP", "CNN", "RNN", "DAE"];
for alg in dlmethods:
if alg in ["ANN", "MLP", "CNN"]:
print ("%s is a feed-forward network." % (alg))
elif alg in ["RNN"]:
print ("%s is a recurrent network." % (alg))
else:
print ("%s is an unsupervised method." % (alg))
print("\nFOR loop with index.")
for alg, i in zip(dlmethods, range(len(dlmethods))):
if alg in ["ANN", "MLP", "CNN"]:
print ("[%d/%d] %s is a feed-forward network."
% (i, len(dlmethods), alg))
elif alg in ["RNN"]:
print ("[%d/%d] %s is a recurrent network."
% (i, len(dlmethods), alg))
else:
print ("[%d/%d] %s is an unsupervised method."
% (i, len(dlmethods), alg))
Note that, index starts with 0 !
Let's make a function in Python
def sum(a, b):
return a+b
X = 10.
Y = 20.
print ("%.1f + %.1f = %.1f" % (X, Y, sum(X, Y)))
10.0 + 20.0 = 30.0
String operations
head = "Deep learning"
body = "very "
tail = "HARD."
print (head + " is " + body + tail)
print (head + " is " + body*3 + tail)
print (head + " is " + body*10 + tail)
print ("\n" + "="*50)
print (" "*15 + "It is used in this way")
print ("="*50 + "\n")
x = "Hello, world"
for i in range(len(x)):
print ("Index: [%02d/%02d] Char: %s"
% (i, len(x), x[i]))
Deep learning is very HARD.
Deep learning is very very very HARD.
Deep learning is very very very very very very very very very very HARD.
==================================================
It is used in this way
==================================================
Index: [00/12] Char: H
Index: [01/12] Char: e
Index: [02/12] Char: l
Index: [03/12] Char: l
Index: [04/12] Char: o
Index: [05/12] Char: ,
Index: [06/12] Char:
Index: [07/12] Char: w
Index: [08/12] Char: o
Index: [09/12] Char: r
Index: [10/12] Char: l
Index: [11/12] Char: d
print ""
idx = -2
print ("(%d)th char is %s" % (idx, x[idx]))
idxfr = 0
idxto = 8
print ("String from %d to %d is [%s]"
% (idxfr, idxto, x[idxfr:idxto]))
idxfr = 4
print ("String from %d to END is [%s]"
% (idxfr, x[idxfr:]))
x = "20160607Cloudy"
year = x[:4]
day = x[4:8]
weather = x[8:]
print ("[%s] -> [%s] + [%s] + [%s] "
% (x, year, day, weather))
(-2)th char is l
String from 0 to 8 is [Hello, w]
String from 4 to END is [o, world]
[20160607Cloudy] -> [2016] + [0607] + [Cloudy]
LIST
a = []
b = [1, 2, 3]
c = ["Hello", ",", "world"]
d = [1, 2, 3, "x", "y", "z"]
x = []
print x
x.append('a')
print x
x.append(123)
print x
x.append(["a", "b"])
print x
print ("Length of x is %d "
% (len(x)))
for i in range(len(x)):
print ("[%02d/%02d] %s"
% (i, len(x), x[i]))
[]
['a']
['a', 123]
['a', 123, ['a', 'b']]
Length of x is 3
[00/03] a
[01/03] 123
[02/03] ['a', 'b']
z = []
z.append(1)
z.append(2)
z.append(3)
z.append('Hello')
for i in range(len(z)):
print (z[i])
1
2
3
Hello
DICTIONARY
dic = dict()
dic["name"] = "Sungjoon"
dic["age"] = 31
dic["job"] = "Ph.D. Candidate"
print dic
{'job': 'Ph.D. Candidate', 'age': 31, 'name': 'Sungjoon'}
Class
class Greeter:
def __init__(self, name):
self.name = name
def greet(self, loud=False):
if loud:
print ('HELLO, %s!'
% self.name.upper())
else:
print ('Hello, %s'
% self.name)
g = Greeter('Fred')
g.greet()
g.greet(loud=True)
Hello, Fred
HELLO, FRED!
def print_np(x):
print ("Type is %s" % (type(x)))
print ("Shape is %s" % (x.shape,))
print ("Values are: \n%s" % (x))
print
RANK 1 ARRAY
x = np.array([1, 2, 3])
print_np(x)
x[0] = 5
print_np(x)
Type is <type 'numpy.ndarray'>
Shape is (3,)
Values are:
[1 2 3]
Type is <type 'numpy.ndarray'>
Shape is (3,)
Values are:
[5 2 3]
RANK 2 ARRAY
y = np.array([[1,2,3], [4,5,6]])
print_np(y)
Type is <type 'numpy.ndarray'>
Shape is (2, 3)
Values are:
[[1 2 3]
[4 5 6]]
ZEROS
a = np.zeros((3, 2))
print_np(a)
Type is <type 'numpy.ndarray'>
Shape is (3, 2)
Values are:
[[ 0. 0.]
[ 0. 0.]
[ 0. 0.]]
ONES
b = np.ones((1, 2))
print_np(b)
Type is <type 'numpy.ndarray'>
Shape is (1, 2)
Values are:
[[ 1. 1.]]
IDENTITY
c = np.eye(2, 2)
print_np(c)
Type is <type 'numpy.ndarray'>
Shape is (2, 2)
Values are:
[[ 1. 0.]
[ 0. 1.]]
d = np.random.random((2, 2))
print_np(d)
Type is <type 'numpy.ndarray'>
Shape is (2, 2)
Values are:
[[ 0.09677829 0.13234216]
[ 0.87168847 0.63200027]]
RANDOM (GAUSSIAN)
e = np.random.randn(1, 10)
print_np(e)
Type is <type 'numpy.ndarray'>
Shape is (1, 10)
Values are:
[[ 1.12732237 -1.50937817 -0.01637454 0.02860102 0.2353765 0.36251934
-1.30868695 -1.16874378 0.8219648 -0.99443059]]
ARRAY INDEXING
a = np.array([[1,2,3,4], [5,6,7,8], [9,10,11,12]])
print_np(a)
print
b = a[:2, 1:3]
print_np(b)
GET ROW
a = np.array([[1,2,3,4], [5,6,7,8], [9,10,11,12]])
print_np(a)
row_r1 = a[1, :]
row_r2 = a[1:2, :]
row_r3 = a[[1], :]
print_np(row_r1)
print_np(row_r2)
print_np(row_r3)
a = np.array([[1,2], [3, 4], [5, 6]])
print_np(a)
b = a[[0, 1, 2], [0, 1, 0]]
print_np(b)
c = np.array([a[0, 0], a[1, 1], a[2, 0]])
print_np(c)
DATATYPES
x = np.array([1, 2])
y = np.array([1.0, 2.0])
z = np.array([1, 2], dtype=np.int64)
print_np(x)
print_np(y)
print_np(z)
Array math
x = np.array([[1,2],[3,4]], dtype=np.float64)
y = np.array([[5,6],[7,8]], dtype=np.float64)
print x + y
print np.add(x, y)
[[ 6. 8.]
[ 10. 12.]]
[[ 6. 8.]
[ 10. 12.]]
print x - y
print np.subtract(x, y)
[[-4. -4.]
[-4. -4.]]
[[-4. -4.]
[-4. -4.]]
print x * y
print np.multiply(x, y)
[[ 5. 12.]
[ 21. 32.]]
[[ 5. 12.]
[ 21. 32.]]
print x / y
print np.divide(x, y)
[[ 0.2 0.33333333]
[ 0.42857143 0.5 ]]
[[ 0.2 0.33333333]
[ 0.42857143 0.5 ]]
print np.sqrt(x)
[[ 1. 1.41421356]
[ 1.73205081 2. ]]
x = np.array([[1,2],[3,4]])
y = np.array([[5,6],[7,8]])
v = np.array([9,10])
w = np.array([11, 12])
print_np(x)
print_np(y)
print_np(v)
print_np(w)
print v.dot(w)
print np.dot(v, w)
print x.dot(v)
print np.dot(x, v)
print x.dot(y)
print np.dot(x, y)
x = np.array([[1,2],[3,4]])
print_np(x)
print
print x
print x.T
print np.sum(x)
print np.sum(x, axis=0)
print np.sum(x, axis=1)
print x
print x.T
v = np.array([1,2,3])
print v
print v.T
v = np.array([[1,2,3]])
print v
print v.T
Other useful operations
x = np.array([[1,2,3], [4,5,6], [7,8,9], [10, 11, 12]])
v = np.array([1, 0, 1])
y = np.empty_like(x)
print_np(x)
print_np(v)
print_np(y)
for i in range(4):
y[i, :] = x[i, :] + v
print_np(y)
vv = np.tile(v, (4, 1))
print_np(vv)
x = np.array([[1,2,3], [4,5,6], [7,8,9], [10, 11, 12]])
v = np.array([1, 0, 1])
y = x + v
print_np(x)
print_np(v)
print_np(y)
x = np.array([[1,2,3], [4,5,6]])
print_np(x)
print_np(v)
print x + v
print_np(x)
print_np(w)
print (x.T + w).T
print
print x + np.reshape(w, (2, 1))
Matplotlib
import matplotlib.pyplot as plt
%matplotlib inline
x = np.arange(0, 3 * np.pi, 0.1)
y = np.sin(x)
plt.plot(x, y)
[<matplotlib.lines.Line2D at 0x7f7bc21b91d0>]
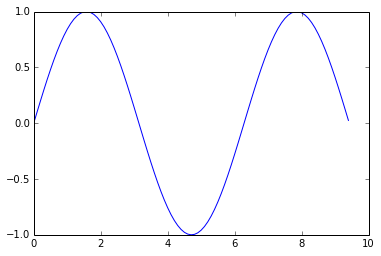
y_sin = np.sin(x)
y_cos = np.cos(x)
plt.plot(x, y_sin)
plt.plot(x, y_cos)
plt.xlabel('x axis label')
plt.ylabel('y axis label')
plt.title('Sine and Cosine')
plt.legend(['Sine', 'Cosine'])
plt.show()
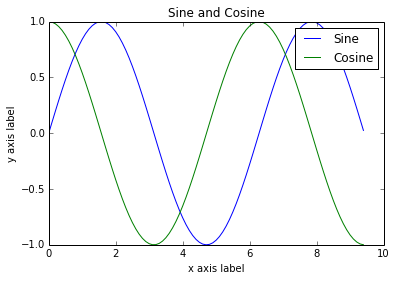
x = np.arange(0, 3 * np.pi, 0.1)
y_sin = np.sin(x)
y_cos = np.cos(x)
plt.subplot(2, 1, 1)
plt.plot(x, y_sin)
plt.title('Sine')
plt.subplot(2, 1, 2)
plt.plot(x, y_cos)
plt.title('Cosine')
plt.show()
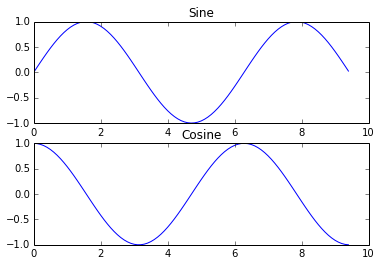